Operation Starfall
Operation Starfall is a 2.5D metroidvania couch Co-op game based on the style of the 80`s Saturday cartoons created by Neon Origins.
Project details:
code language : C#
Software : Unity engine, GitHub and Trello
Team size : 23
My role : Developer and Git master
Date : 13 February 2023 - today
NOTE : GitHub and Trello are private
My Contribution
For the most time I help creating features for in the game but I also helped with managing the GitHub together with the technical director.
Scene creator tool
Scene tool
This is a tool that Is created to make the lives easier for the level designers. With this tool you can create new scenes for the project. Within this tool you can do two things. You can select a name for your scene and select the folder where the scene will be stored.
When you click on select folder a popup will appear. Within this popup are all the folders inside a folder that you have selected to be the root folder. After, you create the scene the system will make the scene, store it in the given folder and also create a duplicate of the terrain data that is used in the scene.
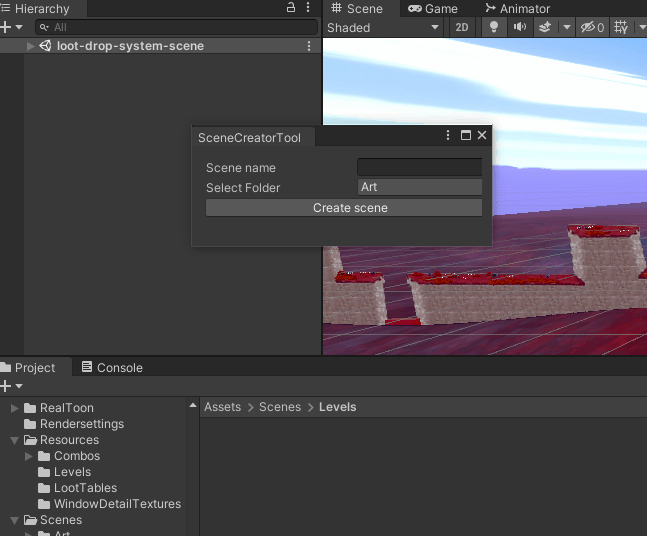
Start creating the new scene
private void StartCreatingNewScene(string assetPath)
{
CopyScene(assetPath);
EditorSceneManager.sceneOpened += OnLoaded;
EditorSceneManager.OpenScene($"{assetPath}/{_name}.unity");
void OnLoaded(Scene createdScene, OpenSceneMode ignoreMe)
{
if (createdScene.name.EndsWith(_staticSceneName)) return;
EditorSceneManager.sceneOpened -= OnLoaded;
var terrains = GetTerrain();
foreach(var terrain in terrains)
CopyTerrainData(terrain);
}
}
private void CopyScene(string path)
{
AssetDatabase.CopyAsset(_templateScenePath, $"{path}/{_name}.unity");
AssetDatabase.CopyAsset(_staticTemplateScenePath, $"{path}/{_name}-{_staticSceneName}.unity");
}
Copy the terrain data
private void CopyTerrainData(Transform terrain)
{
var colliderComponent = terrain.GetComponent<TerrainCollider>();
var terrainComponent = terrain.GetComponent<Terrain>();
var terrainDataPath = AssetDatabase.GetAssetPath(colliderComponent.terrainData);
AssetDatabase.CopyAsset(terrainDataPath, terrainDataPath + "-" +_name + "-" + "TerrainData.asset");
var newTerrainData = AssetDatabase.LoadAssetAtPath<TerrainData>(terrainDataPath + "-" +_name + "-" + "TerrainData.asset");
colliderComponent.terrainData = newTerrainData;
terrainComponent.terrainData = newTerrainData;
}
private List<Transform> GetTerrain()
{
var listOfTerrains = new List<Transform>();
var levelVisuals = GameObject.Find("SaveableObjects").transform.Find("level_visuals");
var terrains = levelVisuals.Find("Terrain").GetComponentsInChildren<Terrain>();
foreach (var terrain in terrains)
{
listOfTerrains.Add(terrain.transform);
}
return listOfTerrains;
}
Get all folders inside the root folder you selected
private string[] GetSceneSubFolders()
{
var folders = GetFolders(_rootFolder).ToArray();
for (var i = 0; i < folders.Length; i++)
{
folders[i] = folders[i].Remove(0, _rootFolder.Length);
folders[i] = folders[i].TrimStart('/');
}
return folders.Where(c => c != "").ToArray();
}
private List<string> GetFolders(string root)
{
var result = new List<string> { root };
result.AddRange(GetSubFolders(root));
return result;
}
private List<string> GetSubFolders(string folder)
{
var subFolders = AssetDatabase.GetSubFolders(folder).ToList();
for (var index = subFolders.Count - 1; index >= 0; index--)
{
var subFolder = subFolders[index];
subFolders.AddRange(GetSubFolders(subFolder));
}
return subFolders;
}
Unique Id
This is a script you can add to any object to give the object a unique id. When you add it to an object it will search for a unique GUID and save it in a variable.
With the id you can quickly find objects in the project. One of the things the id is used for is the character customization.
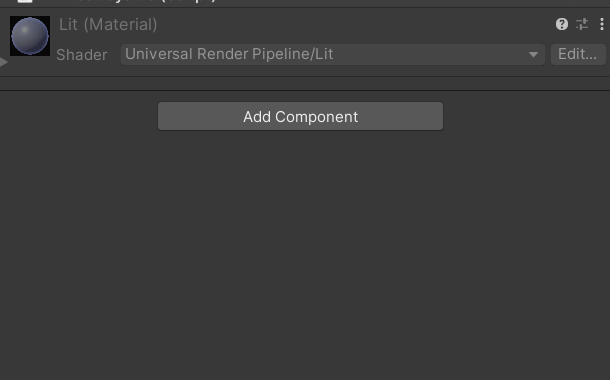
Generate unique id
private void OnValidate()
{
if (hasGeneratedId) return;
GenerateId();
}
private void GenerateId()
{
hasGeneratedId = true;
uniqueId = System.Guid.NewGuid().ToString();
}